In the world of distributed systems and microservices, the ability to communicate effectively between different components of an application is essential. This is where message brokers like RabbitMQ come in. RabbitMQ helps facilitate asynchronous communication, making applications more scalable and decoupled. In this guide, we’ll dive into what RabbitMQ is, how to set it up quickly using Docker, and provide example usage in both JavaScript and C#.
What is RabbitMQ?
RabbitMQ is a message broker that implements the Advanced Message Queuing Protocol (AMQP). In simple terms, it allows different parts of an application to communicate with each other by sending and receiving messages through a queue. Here’s why RabbitMQ is widely used:
- Scalability: RabbitMQ decouples services, making it easier to scale different parts of your system independently.
- Reliability: It provides guaranteed message delivery, ensuring that important data isn’t lost.
- Flexibility: RabbitMQ supports multiple messaging patterns such as direct messaging, publish-subscribe, and more.
With these benefits, RabbitMQ is popular in many use cases, including real-time data processing, background job handling, and more.
How RabbitMQ Works
RabbitMQ works by organizing communication around three core components:
- Producer: The component that sends messages to the queue.
- Queue: The storage unit that holds messages until they are processed.
- Consumer: The component that receives and processes messages from the queue.
Messages are routed to queues by exchanges using routing rules. RabbitMQ supports different types of exchanges, including direct, fanout, topic, and headers, each designed for specific routing patterns.
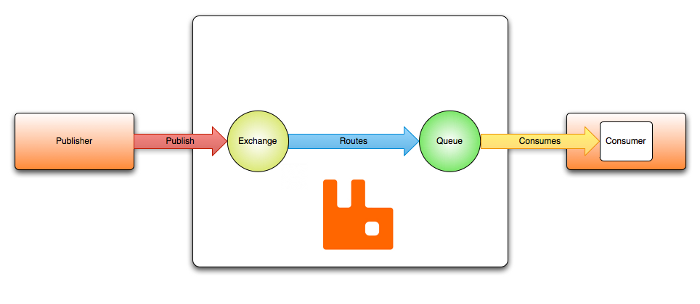
Quick Deployment of RabbitMQ Using Docker and Docker Compose
Setting up RabbitMQ manually can be time-consuming, especially for testing and development purposes. Docker and Docker Compose simplify the process by allowing us to run RabbitMQ with just a few commands. Here’s how:
Step 1: Install Docker and Docker Compose
Before we start, ensure Docker and Docker Compose are installed. If not, you can download them from the official Docker website.
Step 2: Write a Docker Compose File
Create a docker-compose.yml
file to define the RabbitMQ service. Here’s an example file:
version: '3'
services:
rabbitmq:
image: rabbitmq:3-management
container_name: rabbitmq
ports:
- "5672:5672" # Default port for RabbitMQ
- "15672:15672" # Management console
environment:
- RABBITMQ_DEFAULT_USER=admin
- RABBITMQ_DEFAULT_PASS=admin
- Image: We’re using the
rabbitmq:3-management
image, which comes with a web-based management console. - Ports:
5672
is the default port for messaging, and15672
is for the management console. - Environment: Setting default username and password.
Step 3: Launch RabbitMQ
In the directory containing docker-compose.yml
, run the following command:
docker-compose up -d
This command will download the RabbitMQ Docker image (if not already present) and start the RabbitMQ container in detached mode. You can verify that RabbitMQ is running by accessing the management console at http://localhost:15672
using the credentials you set.
Using RabbitMQ in JavaScript (Node.js)
Let’s see how to work with RabbitMQ in JavaScript using the amqplib
library, which provides an easy interface to interact with RabbitMQ.
Install amqplib:
npm install amqplib
Producer Example:The producer sends messages to a queue. Here’s a simple producer that sends a “Hello, RabbitMQ!” message.
const amqp = require('amqplib');
async function sendMessage() {
const connection = await amqp.connect('amqp://localhost');
const channel = await connection.createChannel();
const queue = 'hello';
await channel.assertQueue(queue, { durable: false });
channel.sendToQueue(queue, Buffer.from('Hello, RabbitMQ!'));
console.log(" [x] Sent 'Hello, RabbitMQ!'");
setTimeout(() => {
connection.close();
process.exit(0);
}, 500);
}
sendMessage().catch(console.error);
Consumer Example:The consumer receives messages from the queue.
const amqp = require('amqplib');
async function receiveMessage() {
const connection = await amqp.connect('amqp://localhost');
const channel = await connection.createChannel();
const queue = 'hello';
await channel.assertQueue(queue, { durable: false });
console.log(" [*] Waiting for messages in %s. To exit press CTRL+C", queue);
channel.consume(queue, (msg) => {
console.log(" [x] Received %s", msg.content.toString());
}, { noAck: true });
}
receiveMessage().catch(console.error);
Using RabbitMQ in C# (.NET)
Now, let’s look at how to use RabbitMQ in C#. For this example, we’ll use the RabbitMQ.Client
package.
Install RabbitMQ.Client:In your .NET project, install the RabbitMQ.Client
package via NuGet:
dotnet add package RabbitMQ.Client
Producer Example:Here’s a C# producer that sends a message to a RabbitMQ queue.
using RabbitMQ.Client;
using System;
using System.Text;
class Program
{
public static void Main()
{
var factory = new ConnectionFactory() { HostName = "localhost" };
using (var connection = factory.CreateConnection())
using (var channel = connection.CreateModel())
{
channel.QueueDeclare(queue: "hello",
durable: false,
exclusive: false,
autoDelete: false,
arguments: null);
string message = "Hello, RabbitMQ!";
var body = Encoding.UTF8.GetBytes(message);
channel.BasicPublish(exchange: "",
routingKey: "hello",
basicProperties: null,
body: body);
Console.WriteLine(" [x] Sent {0}", message);
}
}
}
Consumer Example:The following C# consumer listens for messages in the same queue.
using RabbitMQ.Client;
using RabbitMQ.Client.Events;
using System;
using System.Text;
class Program
{
public static void Main()
{
var factory = new ConnectionFactory() { HostName = "localhost" };
using (var connection = factory.CreateConnection())
using (var channel = connection.CreateModel())
{
channel.QueueDeclare(queue: "hello",
durable: false,
exclusive: false,
autoDelete: false,
arguments: null);
Console.WriteLine(" [*] Waiting for messages.");
var consumer = new EventingBasicConsumer(channel);
consumer.Received += (model, ea) =>
{
var body = ea.Body.ToArray();
var message = Encoding.UTF8.GetString(body);
Console.WriteLine(" [x] Received {0}", message);
};
channel.BasicConsume(queue: "hello",
autoAck: true,
consumer: consumer);
Console.WriteLine(" Press [enter] to exit.");
Console.ReadLine();
}
}
}
Conclusion
RabbitMQ is an incredibly useful tool for creating scalable, reliable, and decoupled applications. By setting it up with Docker and Docker Compose, you can get RabbitMQ running quickly and start experimenting with messaging. Whether you’re using JavaScript or C#, RabbitMQ’s cross-language support makes it easy to integrate into your applications.
With this guide, you’re now equipped to implement RabbitMQ and start building messaging-based architectures. Happy coding!
Explore more fascinating blog posts on our site!