In today’s high-speed digital landscape, fast data retrieval and processing are crucial to delivering seamless user experiences. This is where Redis, an in-memory data structure store, comes in. Known for its lightning-fast speed and flexibility, Redis has become a popular choice for caching, session management, job queues, and more. Whether you’re a backend developer looking to boost your application’s performance or someone keen to learn about cutting-edge databases, Redis has much to offer.
What is Redis?
Redis, short for Remote Dictionary Server, is an open-source, NoSQL database primarily used as an in-memory key-value store. Redis holds data directly in RAM, which makes it extremely fast compared to traditional disk-based databases. This speed advantage is critical for applications that require real-time data processing, such as caching, real-time analytics, and gaming leaderboards.
Redis supports various data structures including strings, hashes, lists, sets, sorted sets, and even geospatial data. This versatility allows Redis to serve as more than just a simple key-value store; it’s also effective for message queuing, publish-subscribe systems, and even as a lightweight primary database for specific use cases.
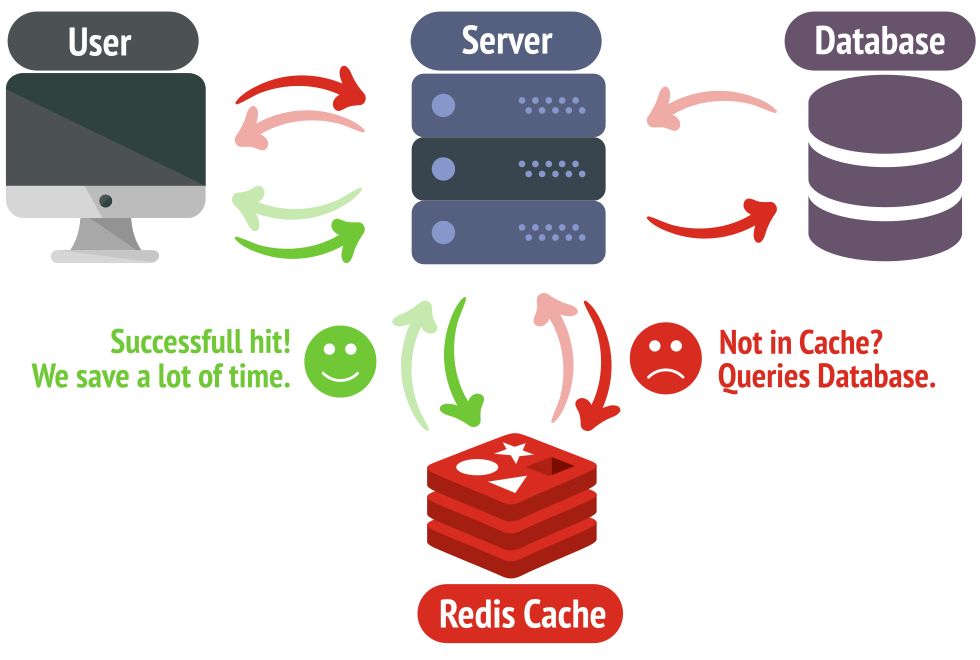
Why Use Redis?
Redis’s in-memory storage and versatile data structures make it a powerful tool for numerous scenarios, particularly those that demand rapid data access and manipulation. Here are some key reasons why developers choose Redis:
- High Performance: With in-memory storage, Redis offers response times in the microsecond range, making it ideal for high-speed applications.
- Supports Multiple Data Types: Redis’s data structures like lists, sets, and sorted sets provide flexibility for diverse use cases.
- Scalability: Redis supports clustering, enabling it to handle high throughput and large datasets while maintaining high performance.
- Persistence Options: Though an in-memory database, Redis offers persistence options for backing up data to disk, ensuring data safety even in case of server restarts.
- Pub/Sub Capabilities: Redis’s publish-subscribe model allows developers to implement real-time messaging systems with ease.
Key Redis Commands for Beginners
Redis commands are straightforward and intuitive. Here’s a quick overview of essential commands:
- SET: Store a value with a specific key.
SET mykey "Hello, Redis!"
- GET: Retrieve the value of a given key.
GET mykey
- DEL: Delete a specific key and its value.
DEL mykey
- EXPIRE: Set an expiration time (in seconds) for a key.
EXPIRE mykey 10
- INCR/DECR: Increment or decrement a value.
INCR mycounter
These commands lay the foundation for using Redis, and they’re straightforward enough to be accessible for Redis beginners.
Redis Use Cases
Redis is highly versatile, making it valuable for a wide range of applications. Here are some of the most common Redis use cases:
1. Caching
Caching frequently accessed data reduces load on the primary database and enhances the speed of web applications. Redis’s in-memory storage makes it a powerful caching tool, allowing developers to store key data (such as user profiles or page content) in Redis for rapid retrieval.
2. Session Management
Redis is often used for session management in web applications. Since Redis stores data in memory, it can handle thousands of simultaneous sessions with very low latency, which is perfect for applications with a high volume of users.
3. Job Queuing
Redis’s lists and sorted sets are great for managing job queues. By pushing tasks onto a list and popping them off as they are processed, Redis enables efficient background processing.
4. Real-time Analytics
With its high-speed data retrieval, Redis is ideal for counting or tracking events in real-time. For example, a news website could use Redis to track the number of visitors per minute, displaying live analytics.
5. Publish-Subscribe Systems
Redis supports the publish-subscribe pattern, which allows one-to-many communication. This is valuable for real-time notifications, chat applications, and streaming data systems where updates need to be broadcast to multiple clients instantly.
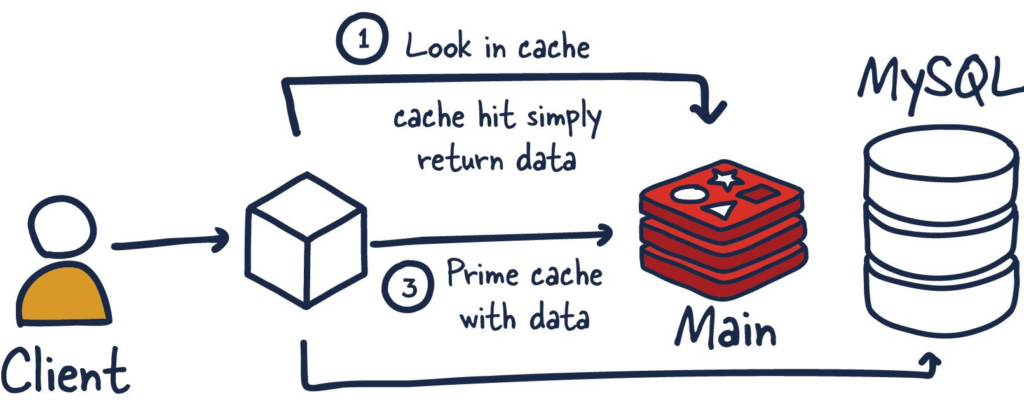
Quickly Deploy Redis with Docker and Docker Compose
Deploying Redis with Docker can simplify setup, especially when working on development environments or in microservices architectures. Docker Compose further streamlines the process by allowing you to configure and manage multiple services with a single file.
1. Running Redis with Docker
If Docker is installed, you can quickly run a Redis container using this command:
docker run --name redis-container -p 6379:6379 -d redis
This command will:
- Pull the latest Redis image from Docker Hub (if not already available locally).
- Run the Redis container in detached mode (
-d
), exposing port6379
(default Redis port).
2. Setting Up Redis with Docker Compose
To manage Redis in a more flexible setup, especially when using multiple containers, Docker Compose can be helpful. Here’s an example docker-compose.yml
file to set up a Redis container:
version: '3'
services:
redis:
image: redis:latest
container_name: redis-container
ports:
- "6379:6379"
volumes:
- redis_data:/data
networks:
- redis_network
volumes:
redis_data:
networks:
redis_network:
Explanation of the Docker Compose File:
- image: Specifies the Redis image to use.
- ports: Maps port
6379
of the container to port6379
of the host. - volumes: Persists data by creating a Docker volume named
redis_data
to store Redis data. - networks: Defines a custom network named
redis_network
to isolate the Redis service (useful for multi-container setups).
Running Docker Compose
Once you have created the docker-compose.yml
file, start Redis with Docker Compose by running:
docker-compose up -d
To stop the Redis container, you can use:
docker-compose down
Using Docker Compose allows you to manage and scale Redis instances easily, making it ideal for development environments and local testing.
Example in JavaScript (Node.js)
Here’s a basic example of using Redis with Node.js, demonstrating how to set and get data.
Install the Redis Client
npm install redis
JavaScript Code
const redis = require("redis");
const client = redis.createClient();
client.on("connect", () => {
console.log("Connected to Redis");
});
// Set a key-value pair
client.set("mykey", "Hello, Redis!", (err, reply) => {
console.log(reply); // Output: OK
});
// Retrieve the value of a key
client.get("mykey", (err, reply) => {
console.log(reply); // Output: Hello, Redis!
});
client.quit();
Example in C#
In C#, the StackExchange.Redis
package is the recommended client for Redis. Here’s a sample C# implementation:
Install StackExchange.Redis
dotnet add package StackExchange.Redis
C# Code
using System;
using StackExchange.Redis;
class Program
{
static void Main()
{
ConnectionMultiplexer redis = ConnectionMultiplexer.Connect("localhost");
IDatabase db = redis.GetDatabase();
// Set a key-value pair
db.StringSet("mykey", "Hello, Redis!");
// Retrieve the value of a key
string value = db.StringGet("mykey");
Console.WriteLine(value); // Output: Hello, Redis!
}
}
Both of these examples highlight how simple and efficient Redis is for storing and retrieving data in high-speed applications.
Conclusion
Redis is a powerful tool in any developer’s toolkit, thanks to its speed, versatility, and ease of use. With applications in caching, session management, job queuing, real-time analytics, and more, Redis serves as a robust solution
Explore more fascinating blog posts on our site!