MongoDB is a powerful NoSQL database solution, and while many developers use it for its flexibility, there are some hidden tricks and optimizations that can make a big difference in your workflows. From running JavaScript within MongoDB to optimizing queries and leveraging advanced features, these tips will help you get the most out of MongoDB and write cleaner, more efficient code.
1. Running JavaScript Code in MongoDB
MongoDB’s shell and aggregation framework support JavaScript, allowing developers to use native JS functions for more complex operations and batch updates. Here’s an example where we use JavaScript to reverse order IDs and update documents in the orders
collection:
db.orders.find({
created_at: {
$gte: ISODate("2024-01-01T00:00:00Z"), // From Jan 1, 2024
$lt: ISODate("2025-01-01T00:00:00Z") // To Dec 31, 2024
}
}).forEach(function(doc) {
let reverse_order_id = doc.order_id.split('').reverse().join('');
// Update document with reverse_order_id
db.orders.updateOne(
{ _id: doc._id },
{ $set: { reverse_order_id: reverse_order_id } }
);
});
In this snippet:
- We filter
orders
based on thecreated_at
date. - For each document, we reverse the
order_id
string and add it back as a new field,reverse_order_id
, to the document.
This approach is powerful for manipulating and updating data in bulk without needing to export or process data outside MongoDB.
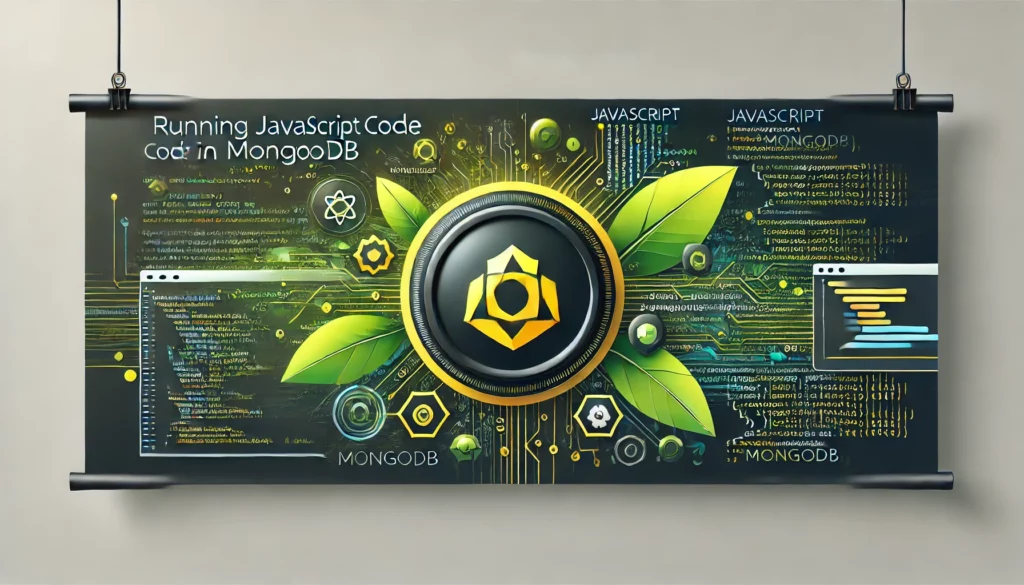
2. Replace Map-Reduce with Aggregation Pipeline
Aggregation Pipeline is a more efficient and often simpler alternative to Map-Reduce for data processing tasks. It allows you to create multi-stage data transformations, such as filtering, grouping, and sorting, within MongoDB. Here’s an example where we calculate the total quantity of products by category:
db.products.aggregate([
{ $group: { _id: "$category", totalQuantity: { $sum: "$quantity" } } }
]);
This aggregation first groups the documents by category
and then sums up the quantity
for each group. Compared to Map-Reduce, Aggregation Pipeline is faster and generally more readable.
3. Use Partial Indexes for Selective Indexing
Partial indexes are a great optimization when you need to index only certain documents within a collection. This selective indexing approach saves storage and improves performance by indexing only documents that meet specific conditions.
For instance, if you only want to index customerId
for active orders in orders
, you can create a partial index:
db.orders.createIndex(
{ customerId: 1 },
{ partialFilterExpression: { status: "active" } }
);
This index will only apply to documents where status
is set to "active"
, resulting in smaller index sizes and faster operations on the collection.
4. Set Up TTL Indexes for Temporary Data
Sometimes, you might have temporary data that doesn’t need to be stored indefinitely, such as session tokens or cache records. A TTL (Time-To-Live) index allows MongoDB to automatically delete documents after a set period.
For example, to automatically delete session documents 24 hours after they are created, you can set up a TTL index on the createdAt
field:
db.sessions.createIndex(
{ createdAt: 1 },
{ expireAfterSeconds: 86400 }
);
This index removes each document in the sessions
collection 24 hours (86400 seconds) after its createdAt
timestamp.
5. Using $merge
to Save Query Results to Another Collection
Sometimes, you may want to store the results of an aggregation in a separate collection for reporting or backup purposes. The $merge
stage in the Aggregation Pipeline allows you to write query results directly to another collection, avoiding the need to process data externally.
Here’s an example where we aggregate daily orders and save the results in a dailyOrders
collection:
db.orders.aggregate([
{ $group: { _id: "$date", totalOrders: { $sum: 1 } } },
{
$merge: {
into: "dailyOrders",
whenMatched: "merge",
whenNotMatched: "insert"
}
}
]);
This aggregation groups orders by date and counts the total orders per day, then inserts or updates these values in the dailyOrders
collection.
6. Use $lookup
for Data Joins Across Collections
MongoDB’s $lookup
stage in the Aggregation Pipeline allows you to join documents from one collection with those from another, much like a SQL join. This is incredibly useful for working with related data across collections.
For example, if you want to add customer information to each order in orders
, you can perform a join with the customers
collection:
db.orders.aggregate([
{
$lookup: {
from: "customers",
localField: "customerId",
foreignField: "_id",
as: "customerInfo"
}
}
]);
This query adds customerInfo
as an array in each order document, containing all customer details for the matching customerId
.
7. Optimize with Projections
Using projections, you can limit the fields returned by a query, reducing both the size of the returned data and the query’s execution time. This is especially useful when querying large collections where you only need a few specific fields.
For instance, to retrieve only the name
and price
fields from products
, you can specify a projection:
db.products.find({}, { name: 1, price: 1 });
Projections like this can make a noticeable difference in performance, especially when dealing with complex documents or high-traffic queries.
8. Leverage $text
and $search
for Full-Text Search
If you need to search text fields in MongoDB, you can enable full-text search by creating a text index on the fields of interest. Text indexes allow you to perform keyword searches within documents, making MongoDB a great option for applications that need simple search functionality.
For example, to search for articles containing “MongoDB” or “NoSQL,” you would first create a text index on the content
field in articles
:
db.articles.createIndex({ content: "text" });
db.articles.find({ $text: { $search: "MongoDB NoSQL" } });
This will return all documents with “MongoDB” or “NoSQL” in the content
field, perfect for adding search capabilities without additional infrastructure.
9. Monitor Changes in Real-Time with Change Streams
MongoDB’s Change Streams provide real-time data updates, allowing you to watch for inserts, updates, deletes, and other operations. This is particularly useful for applications that need live data, like dashboards or real-time notifications.
Here’s how to watch for changes in the orders
collection:
db.orders.watch().on("change", data => {
console.log("Order changed:", data);
});
With Change Streams, you can set up notifications, trigger functions, or simply log changes as they happen, creating dynamic, real-time applications with MongoDB as your backend.
Final Thoughts
With MongoDB, the right tools and techniques make a big difference in both performance and ease of use. Whether you’re optimizing query times with selective indexes, aggregating data with pipelines, or using Change Streams for real-time updates, these tips will help you go beyond MongoDB’s basic capabilities.
Explore these tips and see how they can simplify your MongoDB operations! Whether you’re just starting or a seasoned pro, MongoDB’s hidden capabilities have a lot to offer.
Explore more fascinating blog posts on our site!